
In this article, we will delve into the concept of using an Advanced Decline Ratio chart to analyze financial data. We will employ Python and some widely-used libraries to read and process the data, followed by creating a band chart to visualize the information. The provided code imports the required libraries and scrapes data from a website. It then uses a list of stocks from the website to download data and store it in a pandas DataFrame.
Importing Libraries:
Begin by importing the necessary libraries:
import pandas as pd
import numpy as np
import yfinance as yf
Loading the Data:
Next, use selenium to scrape data from the website:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from bs4 import BeautifulSoup
import time
import requests
from selenium.webdriver.common.action_chains import ActionChains
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.support import expected_conditions as EC
# Create a new instance of the Firefox driver
driver = webdriver.Chrome(ChromeDriverManager().install())
# Navigate to the webpage URL
url = "https://www.set.or.th/th/market/index/set50/overview"
driver.get(url)
# Wait for the page to load
time.sleep(5)
# Find the second table on the page
table = None
while table is None:
soup = BeautifulSoup(driver.page_source, "html.parser")
tables = soup.find_all("table", {"class": "table b-table table-custom-field table-custom-field--cnc table-hover-underline b-table-no-border-collapse b-table-selectable b-table-select-multi"})
# Scroll down the page to load more data
actions = ActionChains(driver)
actions.send_keys(Keys.PAGE_DOWN).perform()
table = tables[0]
# Extract the table headers
headers = [th.text.strip() for th in table.select("thead th")]
# Extract the table rows
rows = []
for tr in table.select("tbody tr"):
rows.append([td.text.strip() for td in tr.select("td")])
# Print the headers and rows of the table
print(headers)
for row in rows:
print(row)
# Close the web driver
driver.quit()
set50_list=rows
result
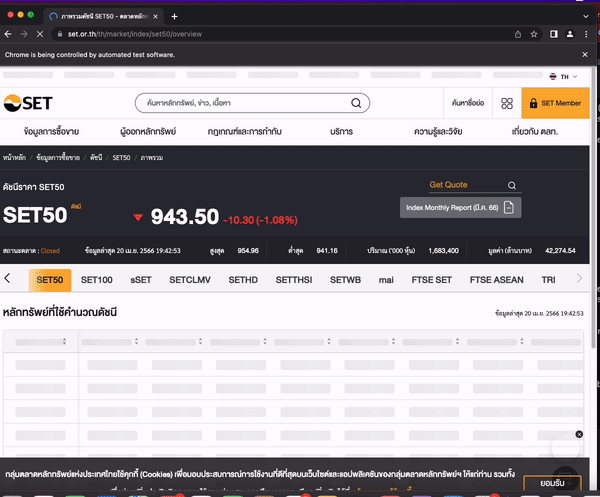
['หลักทรัพย์\n (Click to sort Ascending)', 'เปิด\n (Click to sort Ascending)', 'สูงสุด\n (Click to sort Ascending)', 'ต่ำสุด\n (Click to sort Ascending)', 'ล่าสุด\n (Click to sort Ascending)', 'เปลี่ยนแปลง\n (Click to sort Ascending)', '% เปลี่ยนแปลง\n (Click to sort Ascending)', 'เสนอซื้อ\n (Click to sort Ascending)', 'เสนอขาย\n (Click to sort Ascending)', 'ปริมาณ (หุ้น)\n (Click to sort Ascending)', "มูลค่า ('000 บาท)\n (Click to sort Ascending)"]
['ADVANC', '211.00', '212.00', '209.00', '210.00', '-2.00', '-0.94', '210.00', '211.00', '4,691,365', '986,973.28']
['AOT', '72.25', '72.75', '72.00', '72.25', '-0.25', '-0.34', '72.00', '72.25', '17,551,455', '1,269,367.13']
['AWC', '5.35', '5.45', '5.30', '5.35', '0.00', '0.00', '5.35', '5.40', '34,728,779', '186,514.97']
['BANPU', '9.65', '9.65', '9.50', '9.50', '-0.10', '-1.04', '9.50', '9.55', '54,118,634', '517,509.20']
['BBL', '158.50', '159.50', '156.50', '158.50', '+0.50', '+0.32', '158.00', '158.50', '9,138,471', '1,443,339.52']
['BDMS', '29.75', '29.75', '29.00', '29.50', '0.00', '0.00', '29.25', '29.50', '57,222,628', '1,675,890.88']
['BEM', '9.00', '9.05', '8.90', '8.90', '-0.15', '-1.66', '8.90', '8.95', '16,640,178', '148,715.88']
['BGRIM', '39.25', '39.25', '38.00', '38.50', '-0.75', '-1.91', '38.25', '38.50', '7,556,798', '289,970.67']
['BH', '241.00', '242.00', '239.00', '242.00', '-1.00', '-0.41', '241.00', '242.00', '1,952,385', '469,645.61']
['BTS', '7.70', '7.80', '7.55', '7.60', '-0.10', '-1.30', '7.55', '7.60', '32,545,775', '248,895.92']
['CBG', '80.25', '81.00', '76.75', '77.25', '-4.00', '-4.92', '77.00', '77.25', '14,004,203', '1,097,867.26']
['CENTEL', '54.25', '55.50', '53.75', '55.00', '+0.75', '+1.38', '54.75', '55.00', '3,049,516', '167,007.28']
['COM7', '28.50', '29.00', '27.75', '28.00', '-0.25', '-0.88', '28.00', '28.25', '10,717,883', '302,077.08']
['CPALL', '64.00', '64.25', '63.50', '63.75', '-0.25', '-0.39', '63.75', '64.00', '11,075,918', '706,749.57']
['CPF', '20.90', '20.90', '20.70', '20.70', '-0.20', '-0.96', '20.70', '20.80', '6,615,716', '137,399.75']
['CPN', '69.00', '70.00', '68.75', '69.00', '-0.25', '-0.36', '69.00', '69.25', '6,480,281', '449,116.05']
['CRC', '43.75', '44.25', '43.50', '43.50', '-0.25', '-0.57', '43.50', '43.75', '5,739,627', '251,292.06']
['DELTA', '966.00', '970.00', '956.00', '960.00', '-12.00', '-1.23', '960.00', '962.00', '470,997', '452,708.58']
['EA', '73.75', '74.00', '72.50', '72.75', '-1.00', '-1.36', '72.50', '72.75', '11,800,814', '861,601.68']
['EGCO', '161.50', '161.50', '158.00', '158.00', '-4.00', '-2.47', '158.00', '158.50', '587,378', '93,394.65']
['GLOBAL', '17.40', '17.40', '16.90', '17.10', '-0.40', '-2.29', '17.00', '17.10', '15,313,248', '262,140.87']
['GPSC', '65.00', '65.00', '63.50', '63.75', '-1.50', '-2.30', '63.75', '64.00', '4,184,165', '267,989.58']
['GULF', '52.75', '53.00', '51.50', '51.50', '-1.50', '-2.83', '51.50', '51.75', '21,681,046', '1,126,171.71']
['HMPRO\n \n XD', '13.90', '14.00', '13.50', '13.50', '-0.50', '-3.57', '13.50', '13.60', '33,904,124', '462,121.80']
['INTUCH', '73.25', '74.25', '73.25', '73.50', '0.00', '0.00', '73.50', '73.75', '2,667,470', '196,586.17']
['IVL', '33.75', '34.00', '32.50', '32.50', '-1.25', '-3.70', '32.50', '32.75', '19,821,754', '653,723.54']
['JMART', '19.80', '20.20', '19.10', '19.20', '-0.40', '-2.04', '19.10', '19.20', '30,470,676', '596,668.01']
['JMT', '39.75', '40.00', '37.75', '38.50', '-0.50', '-1.28', '38.50', '38.75', '17,822,985', '692,730.90']
['KBANK', '129.00', '129.50', '125.00', '126.00', '-5.50', '-4.18', '125.50', '126.00', '57,355,933', '7,285,204.57']
['KTB', '17.40', '17.80', '17.40', '17.50', '+0.80', '+4.79', '17.50', '17.60', '284,130,167', '4,999,397.47']
['KTC', '53.75', '54.50', '53.50', '53.75', '0.00', '0.00', '53.75', '54.00', '3,268,631', '176,450.93']
['LH', '9.75', '9.80', '9.70', '9.80', '0.00', '0.00', '9.75', '9.80', '14,502,228', '141,680.20']
['MINT', '31.25', '31.75', '30.75', '31.50', '+0.25', '+0.80', '31.25', '31.50', '16,338,391', '512,245.18']
['MTC', '36.75', '36.75', '36.00', '36.00', '-0.75', '-2.04', '36.00', '36.25', '7,055,923', '255,984.38']
['OR', '22.20', '22.20', '21.70', '22.00', '-0.20', '-0.90', '21.90', '22.00', '38,717,565', '849,101.21']
['OSP', '28.25', '28.50', '27.25', '27.75', '-0.50', '-1.77', '27.50', '27.75', '13,510,037', '374,625.02']
['PTT', '31.00', '31.25', '30.50', '30.50', '-0.50', '-1.61', '30.50', '30.75', '32,000,973', '986,331.55']
['PTTEP', '157.00', '157.00', '153.50', '153.50', '-4.50', '-2.85', '153.50', '154.00', '10,036,777', '1,554,886.07']
['PTTGC', '42.00', '42.25', '40.75', '41.00', '-1.00', '-2.38', '41.00', '41.25', '15,889,895', '654,974.23']
['RATCH', '38.50', '38.75', '38.00', '38.25', '-0.50', '-1.29', '38.00', '38.25', '2,721,599', '104,424.28']
['SAWAD', '55.75', '56.50', '55.25', '56.00', '+0.25', '+0.45', '55.75', '56.00', '4,122,576', '230,629.29']
['SCB', '100.00', '100.50', '98.75', '99.75', '-0.75', '-0.75', '99.75', '100.00', '36,607,662', '3,638,772.69']
['SCC', '311.00', '312.00', '305.00', '305.00', '-4.00', '-1.29', '305.00', '306.00', '1,841,381', '565,428.88']
['SCGP', '43.75', '44.00', '43.25', '43.50', '0.00', '0.00', '43.25', '43.50', '5,902,257', '257,442.65']
['TIDLOR\n \n XD', '23.10', '23.20', '22.80', '22.90', '-0.30', '-1.29', '22.90', '23.00', '13,315,404', '305,958.49']
['TISCO', '99.75', '100.50', '99.50', '99.75', '0.00', '0.00', '99.50', '99.75', '11,325,175', '1,130,895.68']
['TOP', '49.75', '50.00', '48.25', '48.75', '-0.75', '-1.52', '48.75', '49.00', '16,190,521', '793,920.35']
['TRUE', '7.90', '8.05', '7.90', '8.00', '+0.05', '+0.63', '7.95', '8.00', '29,574,578', '235,550.94']
['TTB', '1.45', '1.46', '1.40', '1.42', '0.00', '0.00', '1.41', '1.42', '591,730,403', '843,745.44']
['TU', '14.00', '14.00', '13.80', '13.80', '-0.30', '-2.13', '13.80', '13.90', '24,019,571', '333,447.59']
dfset50 =pd.DataFrame(set50_list)
dfset50
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | ADVANC | 211.00 | 212.00 | 209.00 | 210.00 | -2.00 | -0.94 | 210.00 | 211.00 | 4,691,365 | 986,973.28 |
1 | AOT | 72.25 | 72.75 | 72.00 | 72.25 | -0.25 | -0.34 | 72.00 | 72.25 | 17,551,455 | 1,269,367.13 |
2 | AWC | 5.35 | 5.45 | 5.30 | 5.35 | 0.00 | 0.00 | 5.35 | 5.40 | 34,728,779 | 186,514.97 |
3 | BANPU | 9.65 | 9.65 | 9.50 | 9.50 | -0.10 | -1.04 | 9.50 | 9.55 | 54,118,634 | 517,509.20 |
4 | BBL | 158.50 | 159.50 | 156.50 | 158.50 | +0.50 | +0.32 | 158.00 | 158.50 | 9,138,471 | 1,443,339.52 |
5 | BDMS | 29.75 | 29.75 | 29.00 | 29.50 | 0.00 | 0.00 | 29.25 | 29.50 | 57,222,628 | 1,675,890.88 |
6 | BEM | 9.00 | 9.05 | 8.90 | 8.90 | -0.15 | -1.66 | 8.90 | 8.95 | 16,640,178 | 148,715.88 |
7 | BGRIM | 39.25 | 39.25 | 38.00 | 38.50 | -0.75 | -1.91 | 38.25 | 38.50 | 7,556,798 | 289,970.67 |
8 | BH | 241.00 | 242.00 | 239.00 | 242.00 | -1.00 | -0.41 | 241.00 | 242.00 | 1,952,385 | 469,645.61 |
9 | BTS | 7.70 | 7.80 | 7.55 | 7.60 | -0.10 | -1.30 | 7.55 | 7.60 | 32,545,775 | 248,895.92 |
10 | CBG | 80.25 | 81.00 | 76.75 | 77.25 | -4.00 | -4.92 | 77.00 | 77.25 | 14,004,203 | 1,097,867.26 |
11 | CENTEL | 54.25 | 55.50 | 53.75 | 55.00 | +0.75 | +1.38 | 54.75 | 55.00 | 3,049,516 | 167,007.28 |
12 | COM7 | 28.50 | 29.00 | 27.75 | 28.00 | -0.25 | -0.88 | 28.00 | 28.25 | 10,717,883 | 302,077.08 |
13 | CPALL | 64.00 | 64.25 | 63.50 | 63.75 | -0.25 | -0.39 | 63.75 | 64.00 | 11,075,918 | 706,749.57 |
14 | CPF | 20.90 | 20.90 | 20.70 | 20.70 | -0.20 | -0.96 | 20.70 | 20.80 | 6,615,716 | 137,399.75 |
15 | CPN | 69.00 | 70.00 | 68.75 | 69.00 | -0.25 | -0.36 | 69.00 | 69.25 | 6,480,281 | 449,116.05 |
16 | CRC | 43.75 | 44.25 | 43.50 | 43.50 | -0.25 | -0.57 | 43.50 | 43.75 | 5,739,627 | 251,292.06 |
17 | DELTA | 966.00 | 970.00 | 956.00 | 960.00 | -12.00 | -1.23 | 960.00 | 962.00 | 470,997 | 452,708.58 |
18 | EA | 73.75 | 74.00 | 72.50 | 72.75 | -1.00 | -1.36 | 72.50 | 72.75 | 11,800,814 | 861,601.68 |
19 | EGCO | 161.50 | 161.50 | 158.00 | 158.00 | -4.00 | -2.47 | 158.00 | 158.50 | 587,378 | 93,394.65 |
20 | GLOBAL | 17.40 | 17.40 | 16.90 | 17.10 | -0.40 | -2.29 | 17.00 | 17.10 | 15,313,248 | 262,140.87 |
21 | GPSC | 65.00 | 65.00 | 63.50 | 63.75 | -1.50 | -2.30 | 63.75 | 64.00 | 4,184,165 | 267,989.58 |
22 | GULF | 52.75 | 53.00 | 51.50 | 51.50 | -1.50 | -2.83 | 51.50 | 51.75 | 21,681,046 | 1,126,171.71 |
23 | HMPRO\n \n XD | 13.90 | 14.00 | 13.50 | 13.50 | -0.50 | -3.57 | 13.50 | 13.60 | 33,904,124 | 462,121.80 |
24 | INTUCH | 73.25 | 74.25 | 73.25 | 73.50 | 0.00 | 0.00 | 73.50 | 73.75 | 2,667,470 | 196,586.17 |
25 | IVL | 33.75 | 34.00 | 32.50 | 32.50 | -1.25 | -3.70 | 32.50 | 32.75 | 19,821,754 | 653,723.54 |
26 | JMART | 19.80 | 20.20 | 19.10 | 19.20 | -0.40 | -2.04 | 19.10 | 19.20 | 30,470,676 | 596,668.01 |
27 | JMT | 39.75 | 40.00 | 37.75 | 38.50 | -0.50 | -1.28 | 38.50 | 38.75 | 17,822,985 | 692,730.90 |
28 | KBANK | 129.00 | 129.50 | 125.00 | 126.00 | -5.50 | -4.18 | 125.50 | 126.00 | 57,355,933 | 7,285,204.57 |
29 | KTB | 17.40 | 17.80 | 17.40 | 17.50 | +0.80 | +4.79 | 17.50 | 17.60 | 284,130,167 | 4,999,397.47 |
30 | KTC | 53.75 | 54.50 | 53.50 | 53.75 | 0.00 | 0.00 | 53.75 | 54.00 | 3,268,631 | 176,450.93 |
31 | LH | 9.75 | 9.80 | 9.70 | 9.80 | 0.00 | 0.00 | 9.75 | 9.80 | 14,502,228 | 141,680.20 |
32 | MINT | 31.25 | 31.75 | 30.75 | 31.50 | +0.25 | +0.80 | 31.25 | 31.50 | 16,338,391 | 512,245.18 |
33 | MTC | 36.75 | 36.75 | 36.00 | 36.00 | -0.75 | -2.04 | 36.00 | 36.25 | 7,055,923 | 255,984.38 |
34 | OR | 22.20 | 22.20 | 21.70 | 22.00 | -0.20 | -0.90 | 21.90 | 22.00 | 38,717,565 | 849,101.21 |
35 | OSP | 28.25 | 28.50 | 27.25 | 27.75 | -0.50 | -1.77 | 27.50 | 27.75 | 13,510,037 | 374,625.02 |
36 | PTT | 31.00 | 31.25 | 30.50 | 30.50 | -0.50 | -1.61 | 30.50 | 30.75 | 32,000,973 | 986,331.55 |
37 | PTTEP | 157.00 | 157.00 | 153.50 | 153.50 | -4.50 | -2.85 | 153.50 | 154.00 | 10,036,777 | 1,554,886.07 |
38 | PTTGC | 42.00 | 42.25 | 40.75 | 41.00 | -1.00 | -2.38 | 41.00 | 41.25 | 15,889,895 | 654,974.23 |
39 | RATCH | 38.50 | 38.75 | 38.00 | 38.25 | -0.50 | -1.29 | 38.00 | 38.25 | 2,721,599 | 104,424.28 |
40 | SAWAD | 55.75 | 56.50 | 55.25 | 56.00 | +0.25 | +0.45 | 55.75 | 56.00 | 4,122,576 | 230,629.29 |
41 | SCB | 100.00 | 100.50 | 98.75 | 99.75 | -0.75 | -0.75 | 99.75 | 100.00 | 36,607,662 | 3,638,772.69 |
42 | SCC | 311.00 | 312.00 | 305.00 | 305.00 | -4.00 | -1.29 | 305.00 | 306.00 | 1,841,381 | 565,428.88 |
43 | SCGP | 43.75 | 44.00 | 43.25 | 43.50 | 0.00 | 0.00 | 43.25 | 43.50 | 5,902,257 | 257,442.65 |
44 | TIDLOR\n \n XD | 23.10 | 23.20 | 22.80 | 22.90 | -0.30 | -1.29 | 22.90 | 23.00 | 13,315,404 | 305,958.49 |
45 | TISCO | 99.75 | 100.50 | 99.50 | 99.75 | 0.00 | 0.00 | 99.50 | 99.75 | 11,325,175 | 1,130,895.68 |
46 | TOP | 49.75 | 50.00 | 48.25 | 48.75 | -0.75 | -1.52 | 48.75 | 49.00 | 16,190,521 | 793,920.35 |
47 | TRUE | 7.90 | 8.05 | 7.90 | 8.00 | +0.05 | +0.63 | 7.95 | 8.00 | 29,574,578 | 235,550.94 |
48 | TTB | 1.45 | 1.46 | 1.40 | 1.42 | 0.00 | 0.00 | 1.41 | 1.42 | 591,730,403 | 843,745.44 |
49 | TU | 14.00 | 14.00 | 13.80 | 13.80 | -0.30 | -2.13 | 13.80 | 13.90 | 24,019,571 | 333,447.59 |
Cleaning Data:
Remove unnecessary characters like ‘\n’ and ‘XD’:
dfset50[0]=dfset50[0].str.replace('\n','')
dfset50[0]=dfset50[0].str.replace('XD','')
dfset50
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | ADVANC | 211.00 | 212.00 | 209.00 | 210.00 | -2.00 | -0.94 | 210.00 | 211.00 | 4,691,365 | 986,973.28 |
1 | AOT | 72.25 | 72.75 | 72.00 | 72.25 | -0.25 | -0.34 | 72.00 | 72.25 | 17,551,455 | 1,269,367.13 |
2 | AWC | 5.35 | 5.45 | 5.30 | 5.35 | 0.00 | 0.00 | 5.35 | 5.40 | 34,728,779 | 186,514.97 |
3 | BANPU | 9.65 | 9.65 | 9.50 | 9.50 | -0.10 | -1.04 | 9.50 | 9.55 | 54,118,634 | 517,509.20 |
4 | BBL | 158.50 | 159.50 | 156.50 | 158.50 | +0.50 | +0.32 | 158.00 | 158.50 | 9,138,471 | 1,443,339.52 |
5 | BDMS | 29.75 | 29.75 | 29.00 | 29.50 | 0.00 | 0.00 | 29.25 | 29.50 | 57,222,628 | 1,675,890.88 |
6 | BEM | 9.00 | 9.05 | 8.90 | 8.90 | -0.15 | -1.66 | 8.90 | 8.95 | 16,640,178 | 148,715.88 |
7 | BGRIM | 39.25 | 39.25 | 38.00 | 38.50 | -0.75 | -1.91 | 38.25 | 38.50 | 7,556,798 | 289,970.67 |
8 | BH | 241.00 | 242.00 | 239.00 | 242.00 | -1.00 | -0.41 | 241.00 | 242.00 | 1,952,385 | 469,645.61 |
9 | BTS | 7.70 | 7.80 | 7.55 | 7.60 | -0.10 | -1.30 | 7.55 | 7.60 | 32,545,775 | 248,895.92 |
10 | CBG | 80.25 | 81.00 | 76.75 | 77.25 | -4.00 | -4.92 | 77.00 | 77.25 | 14,004,203 | 1,097,867.26 |
11 | CENTEL | 54.25 | 55.50 | 53.75 | 55.00 | +0.75 | +1.38 | 54.75 | 55.00 | 3,049,516 | 167,007.28 |
12 | COM7 | 28.50 | 29.00 | 27.75 | 28.00 | -0.25 | -0.88 | 28.00 | 28.25 | 10,717,883 | 302,077.08 |
13 | CPALL | 64.00 | 64.25 | 63.50 | 63.75 | -0.25 | -0.39 | 63.75 | 64.00 | 11,075,918 | 706,749.57 |
14 | CPF | 20.90 | 20.90 | 20.70 | 20.70 | -0.20 | -0.96 | 20.70 | 20.80 | 6,615,716 | 137,399.75 |
15 | CPN | 69.00 | 70.00 | 68.75 | 69.00 | -0.25 | -0.36 | 69.00 | 69.25 | 6,480,281 | 449,116.05 |
16 | CRC | 43.75 | 44.25 | 43.50 | 43.50 | -0.25 | -0.57 | 43.50 | 43.75 | 5,739,627 | 251,292.06 |
17 | DELTA | 966.00 | 970.00 | 956.00 | 960.00 | -12.00 | -1.23 | 960.00 | 962.00 | 470,997 | 452,708.58 |
18 | EA | 73.75 | 74.00 | 72.50 | 72.75 | -1.00 | -1.36 | 72.50 | 72.75 | 11,800,814 | 861,601.68 |
19 | EGCO | 161.50 | 161.50 | 158.00 | 158.00 | -4.00 | -2.47 | 158.00 | 158.50 | 587,378 | 93,394.65 |
20 | GLOBAL | 17.40 | 17.40 | 16.90 | 17.10 | -0.40 | -2.29 | 17.00 | 17.10 | 15,313,248 | 262,140.87 |
21 | GPSC | 65.00 | 65.00 | 63.50 | 63.75 | -1.50 | -2.30 | 63.75 | 64.00 | 4,184,165 | 267,989.58 |
22 | GULF | 52.75 | 53.00 | 51.50 | 51.50 | -1.50 | -2.83 | 51.50 | 51.75 | 21,681,046 | 1,126,171.71 |
23 | HMPRO | 13.90 | 14.00 | 13.50 | 13.50 | -0.50 | -3.57 | 13.50 | 13.60 | 33,904,124 | 462,121.80 |
24 | INTUCH | 73.25 | 74.25 | 73.25 | 73.50 | 0.00 | 0.00 | 73.50 | 73.75 | 2,667,470 | 196,586.17 |
25 | IVL | 33.75 | 34.00 | 32.50 | 32.50 | -1.25 | -3.70 | 32.50 | 32.75 | 19,821,754 | 653,723.54 |
26 | JMART | 19.80 | 20.20 | 19.10 | 19.20 | -0.40 | -2.04 | 19.10 | 19.20 | 30,470,676 | 596,668.01 |
27 | JMT | 39.75 | 40.00 | 37.75 | 38.50 | -0.50 | -1.28 | 38.50 | 38.75 | 17,822,985 | 692,730.90 |
28 | KBANK | 129.00 | 129.50 | 125.00 | 126.00 | -5.50 | -4.18 | 125.50 | 126.00 | 57,355,933 | 7,285,204.57 |
29 | KTB | 17.40 | 17.80 | 17.40 | 17.50 | +0.80 | +4.79 | 17.50 | 17.60 | 284,130,167 | 4,999,397.47 |
30 | KTC | 53.75 | 54.50 | 53.50 | 53.75 | 0.00 | 0.00 | 53.75 | 54.00 | 3,268,631 | 176,450.93 |
31 | LH | 9.75 | 9.80 | 9.70 | 9.80 | 0.00 | 0.00 | 9.75 | 9.80 | 14,502,228 | 141,680.20 |
32 | MINT | 31.25 | 31.75 | 30.75 | 31.50 | +0.25 | +0.80 | 31.25 | 31.50 | 16,338,391 | 512,245.18 |
33 | MTC | 36.75 | 36.75 | 36.00 | 36.00 | -0.75 | -2.04 | 36.00 | 36.25 | 7,055,923 | 255,984.38 |
34 | OR | 22.20 | 22.20 | 21.70 | 22.00 | -0.20 | -0.90 | 21.90 | 22.00 | 38,717,565 | 849,101.21 |
35 | OSP | 28.25 | 28.50 | 27.25 | 27.75 | -0.50 | -1.77 | 27.50 | 27.75 | 13,510,037 | 374,625.02 |
36 | PTT | 31.00 | 31.25 | 30.50 | 30.50 | -0.50 | -1.61 | 30.50 | 30.75 | 32,000,973 | 986,331.55 |
37 | PTTEP | 157.00 | 157.00 | 153.50 | 153.50 | -4.50 | -2.85 | 153.50 | 154.00 | 10,036,777 | 1,554,886.07 |
38 | PTTGC | 42.00 | 42.25 | 40.75 | 41.00 | -1.00 | -2.38 | 41.00 | 41.25 | 15,889,895 | 654,974.23 |
39 | RATCH | 38.50 | 38.75 | 38.00 | 38.25 | -0.50 | -1.29 | 38.00 | 38.25 | 2,721,599 | 104,424.28 |
40 | SAWAD | 55.75 | 56.50 | 55.25 | 56.00 | +0.25 | +0.45 | 55.75 | 56.00 | 4,122,576 | 230,629.29 |
41 | SCB | 100.00 | 100.50 | 98.75 | 99.75 | -0.75 | -0.75 | 99.75 | 100.00 | 36,607,662 | 3,638,772.69 |
42 | SCC | 311.00 | 312.00 | 305.00 | 305.00 | -4.00 | -1.29 | 305.00 | 306.00 | 1,841,381 | 565,428.88 |
43 | SCGP | 43.75 | 44.00 | 43.25 | 43.50 | 0.00 | 0.00 | 43.25 | 43.50 | 5,902,257 | 257,442.65 |
44 | TIDLOR | 23.10 | 23.20 | 22.80 | 22.90 | -0.30 | -1.29 | 22.90 | 23.00 | 13,315,404 | 305,958.49 |
45 | TISCO | 99.75 | 100.50 | 99.50 | 99.75 | 0.00 | 0.00 | 99.50 | 99.75 | 11,325,175 | 1,130,895.68 |
46 | TOP | 49.75 | 50.00 | 48.25 | 48.75 | -0.75 | -1.52 | 48.75 | 49.00 | 16,190,521 | 793,920.35 |
47 | TRUE | 7.90 | 8.05 | 7.90 | 8.00 | +0.05 | +0.63 | 7.95 | 8.00 | 29,574,578 | 235,550.94 |
48 | TTB | 1.45 | 1.46 | 1.40 | 1.42 | 0.00 | 0.00 | 1.41 | 1.42 | 591,730,403 | 843,745.44 |
49 | TU | 14.00 | 14.00 | 13.80 | 13.80 | -0.30 | -2.13 | 13.80 | 13.90 | 24,019,571 | 333,447.59 |
Set column name ‘symbol’ to column 0:
dfset50 = dfset50.rename(columns={0: 'symbol'})
dfset50
symbol | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | ADVANC | 211.00 | 212.00 | 209.00 | 210.00 | -2.00 | -0.94 | 210.00 | 211.00 | 4,691,365 | 986,973.28 |
1 | AOT | 72.25 | 72.75 | 72.00 | 72.25 | -0.25 | -0.34 | 72.00 | 72.25 | 17,551,455 | 1,269,367.13 |
2 | AWC | 5.35 | 5.45 | 5.30 | 5.35 | 0.00 | 0.00 | 5.35 | 5.40 | 34,728,779 | 186,514.97 |
3 | BANPU | 9.65 | 9.65 | 9.50 | 9.50 | -0.10 | -1.04 | 9.50 | 9.55 | 54,118,634 | 517,509.20 |
4 | BBL | 158.50 | 159.50 | 156.50 | 158.50 | +0.50 | +0.32 | 158.00 | 158.50 | 9,138,471 | 1,443,339.52 |
5 | BDMS | 29.75 | 29.75 | 29.00 | 29.50 | 0.00 | 0.00 | 29.25 | 29.50 | 57,222,628 | 1,675,890.88 |
6 | BEM | 9.00 | 9.05 | 8.90 | 8.90 | -0.15 | -1.66 | 8.90 | 8.95 | 16,640,178 | 148,715.88 |
7 | BGRIM | 39.25 | 39.25 | 38.00 | 38.50 | -0.75 | -1.91 | 38.25 | 38.50 | 7,556,798 | 289,970.67 |
8 | BH | 241.00 | 242.00 | 239.00 | 242.00 | -1.00 | -0.41 | 241.00 | 242.00 | 1,952,385 | 469,645.61 |
9 | BTS | 7.70 | 7.80 | 7.55 | 7.60 | -0.10 | -1.30 | 7.55 | 7.60 | 32,545,775 | 248,895.92 |
10 | CBG | 80.25 | 81.00 | 76.75 | 77.25 | -4.00 | -4.92 | 77.00 | 77.25 | 14,004,203 | 1,097,867.26 |
11 | CENTEL | 54.25 | 55.50 | 53.75 | 55.00 | +0.75 | +1.38 | 54.75 | 55.00 | 3,049,516 | 167,007.28 |
12 | COM7 | 28.50 | 29.00 | 27.75 | 28.00 | -0.25 | -0.88 | 28.00 | 28.25 | 10,717,883 | 302,077.08 |
13 | CPALL | 64.00 | 64.25 | 63.50 | 63.75 | -0.25 | -0.39 | 63.75 | 64.00 | 11,075,918 | 706,749.57 |
14 | CPF | 20.90 | 20.90 | 20.70 | 20.70 | -0.20 | -0.96 | 20.70 | 20.80 | 6,615,716 | 137,399.75 |
15 | CPN | 69.00 | 70.00 | 68.75 | 69.00 | -0.25 | -0.36 | 69.00 | 69.25 | 6,480,281 | 449,116.05 |
16 | CRC | 43.75 | 44.25 | 43.50 | 43.50 | -0.25 | -0.57 | 43.50 | 43.75 | 5,739,627 | 251,292.06 |
17 | DELTA | 966.00 | 970.00 | 956.00 | 960.00 | -12.00 | -1.23 | 960.00 | 962.00 | 470,997 | 452,708.58 |
18 | EA | 73.75 | 74.00 | 72.50 | 72.75 | -1.00 | -1.36 | 72.50 | 72.75 | 11,800,814 | 861,601.68 |
19 | EGCO | 161.50 | 161.50 | 158.00 | 158.00 | -4.00 | -2.47 | 158.00 | 158.50 | 587,378 | 93,394.65 |
20 | GLOBAL | 17.40 | 17.40 | 16.90 | 17.10 | -0.40 | -2.29 | 17.00 | 17.10 | 15,313,248 | 262,140.87 |
21 | GPSC | 65.00 | 65.00 | 63.50 | 63.75 | -1.50 | -2.30 | 63.75 | 64.00 | 4,184,165 | 267,989.58 |
22 | GULF | 52.75 | 53.00 | 51.50 | 51.50 | -1.50 | -2.83 | 51.50 | 51.75 | 21,681,046 | 1,126,171.71 |
23 | HMPRO | 13.90 | 14.00 | 13.50 | 13.50 | -0.50 | -3.57 | 13.50 | 13.60 | 33,904,124 | 462,121.80 |
24 | INTUCH | 73.25 | 74.25 | 73.25 | 73.50 | 0.00 | 0.00 | 73.50 | 73.75 | 2,667,470 | 196,586.17 |
25 | IVL | 33.75 | 34.00 | 32.50 | 32.50 | -1.25 | -3.70 | 32.50 | 32.75 | 19,821,754 | 653,723.54 |
26 | JMART | 19.80 | 20.20 | 19.10 | 19.20 | -0.40 | -2.04 | 19.10 | 19.20 | 30,470,676 | 596,668.01 |
27 | JMT | 39.75 | 40.00 | 37.75 | 38.50 | -0.50 | -1.28 | 38.50 | 38.75 | 17,822,985 | 692,730.90 |
28 | KBANK | 129.00 | 129.50 | 125.00 | 126.00 | -5.50 | -4.18 | 125.50 | 126.00 | 57,355,933 | 7,285,204.57 |
29 | KTB | 17.40 | 17.80 | 17.40 | 17.50 | +0.80 | +4.79 | 17.50 | 17.60 | 284,130,167 | 4,999,397.47 |
30 | KTC | 53.75 | 54.50 | 53.50 | 53.75 | 0.00 | 0.00 | 53.75 | 54.00 | 3,268,631 | 176,450.93 |
31 | LH | 9.75 | 9.80 | 9.70 | 9.80 | 0.00 | 0.00 | 9.75 | 9.80 | 14,502,228 | 141,680.20 |
32 | MINT | 31.25 | 31.75 | 30.75 | 31.50 | +0.25 | +0.80 | 31.25 | 31.50 | 16,338,391 | 512,245.18 |
33 | MTC | 36.75 | 36.75 | 36.00 | 36.00 | -0.75 | -2.04 | 36.00 | 36.25 | 7,055,923 | 255,984.38 |
34 | OR | 22.20 | 22.20 | 21.70 | 22.00 | -0.20 | -0.90 | 21.90 | 22.00 | 38,717,565 | 849,101.21 |
35 | OSP | 28.25 | 28.50 | 27.25 | 27.75 | -0.50 | -1.77 | 27.50 | 27.75 | 13,510,037 | 374,625.02 |
36 | PTT | 31.00 | 31.25 | 30.50 | 30.50 | -0.50 | -1.61 | 30.50 | 30.75 | 32,000,973 | 986,331.55 |
37 | PTTEP | 157.00 | 157.00 | 153.50 | 153.50 | -4.50 | -2.85 | 153.50 | 154.00 | 10,036,777 | 1,554,886.07 |
38 | PTTGC | 42.00 | 42.25 | 40.75 | 41.00 | -1.00 | -2.38 | 41.00 | 41.25 | 15,889,895 | 654,974.23 |
39 | RATCH | 38.50 | 38.75 | 38.00 | 38.25 | -0.50 | -1.29 | 38.00 | 38.25 | 2,721,599 | 104,424.28 |
40 | SAWAD | 55.75 | 56.50 | 55.25 | 56.00 | +0.25 | +0.45 | 55.75 | 56.00 | 4,122,576 | 230,629.29 |
41 | SCB | 100.00 | 100.50 | 98.75 | 99.75 | -0.75 | -0.75 | 99.75 | 100.00 | 36,607,662 | 3,638,772.69 |
42 | SCC | 311.00 | 312.00 | 305.00 | 305.00 | -4.00 | -1.29 | 305.00 | 306.00 | 1,841,381 | 565,428.88 |
43 | SCGP | 43.75 | 44.00 | 43.25 | 43.50 | 0.00 | 0.00 | 43.25 | 43.50 | 5,902,257 | 257,442.65 |
44 | TIDLOR | 23.10 | 23.20 | 22.80 | 22.90 | -0.30 | -1.29 | 22.90 | 23.00 | 13,315,404 | 305,958.49 |
45 | TISCO | 99.75 | 100.50 | 99.50 | 99.75 | 0.00 | 0.00 | 99.50 | 99.75 | 11,325,175 | 1,130,895.68 |
46 | TOP | 49.75 | 50.00 | 48.25 | 48.75 | -0.75 | -1.52 | 48.75 | 49.00 | 16,190,521 | 793,920.35 |
47 | TRUE | 7.90 | 8.05 | 7.90 | 8.00 | +0.05 | +0.63 | 7.95 | 8.00 | 29,574,578 | 235,550.94 |
48 | TTB | 1.45 | 1.46 | 1.40 | 1.42 | 0.00 | 0.00 | 1.41 | 1.42 | 591,730,403 | 843,745.44 |
49 | TU | 14.00 | 14.00 | 13.80 | 13.80 | -0.30 | -2.13 | 13.80 | 13.90 | 24,019,571 | 333,447.59 |
Add ‘.BK’ to the ‘symbol’ column to download data from yfinance:
dfset50=dfset50['symbol']+'.BK'
dfset50
0 ADVANC.BK
1 AOT.BK
2 AWC.BK
3 BANPU.BK
4 BBL.BK
5 BDMS.BK
6 BEM.BK
7 BGRIM.BK
8 BH.BK
9 BTS.BK
10 CBG.BK
11 CENTEL.BK
12 COM7.BK
13 CPALL.BK
14 CPF.BK
15 CPN.BK
16 CRC.BK
17 DELTA.BK
18 EA.BK
19 EGCO.BK
20 GLOBAL.BK
21 GPSC.BK
22 GULF.BK
23 HMPRO .BK
24 INTUCH.BK
25 IVL.BK
26 JMART.BK
27 JMT.BK
28 KBANK.BK
29 KTB.BK
30 KTC.BK
31 LH.BK
32 MINT.BK
33 MTC.BK
34 OR.BK
35 OSP.BK
36 PTT.BK
37 PTTEP.BK
38 PTTGC.BK
39 RATCH.BK
40 SAWAD.BK
41 SCB.BK
42 SCC.BK
43 SCGP.BK
44 TIDLOR .BK
45 TISCO.BK
46 TOP.BK
47 TRUE.BK
48 TTB.BK
49 TU.BK
Name: symbol, dtype: object
dfset50=pd.DataFrame(dfset50)
symbol_list_set50 = dfset50['symbol'].tolist()
symbol_list_set50
['ADVANC.BK',
'AOT.BK',
'AWC.BK',
'BANPU.BK',
'BBL.BK',
'BDMS.BK',
'BEM.BK',
'BGRIM.BK',
'BH.BK',
'BTS.BK',
'CBG.BK',
'CENTEL.BK',
'COM7.BK',
'CPALL.BK',
'CPF.BK',
'CPN.BK',
'CRC.BK',
'DELTA.BK',
'EA.BK',
'EGCO.BK',
'GLOBAL.BK',
'GPSC.BK',
'GULF.BK',
'HMPRO .BK',
'INTUCH.BK',
'IVL.BK',
'JMART.BK',
'JMT.BK',
'KBANK.BK',
'KTB.BK',
'KTC.BK',
'LH.BK',
'MINT.BK',
'MTC.BK',
'OR.BK',
'OSP.BK',
'PTT.BK',
'PTTEP.BK',
'PTTGC.BK',
'RATCH.BK',
'SAWAD.BK',
'SCB.BK',
'SCC.BK',
'SCGP.BK',
'TIDLOR .BK',
'TISCO.BK',
'TOP.BK',
'TRUE.BK',
'TTB.BK',
'TU.BK']
Clean data to remove white space:
symbol_list_set50 = [symbol.replace(' ', '') for symbol in symbol_list_set50]
symbol_list_set50
['ADVANC.BK',
'AOT.BK',
'AWC.BK',
'BANPU.BK',
'BBL.BK',
'BDMS.BK',
'BEM.BK',
'BGRIM.BK',
'BH.BK',
'BTS.BK',
'CBG.BK',
'CENTEL.BK',
'COM7.BK',
'CPALL.BK',
'CPF.BK',
'CPN.BK',
'CRC.BK',
'DELTA.BK',
'EA.BK',
'EGCO.BK',
'GLOBAL.BK',
'GPSC.BK',
'GULF.BK',
'HMPRO.BK',
'INTUCH.BK',
'IVL.BK',
'JMART.BK',
'JMT.BK',
'KBANK.BK',
'KTB.BK',
'KTC.BK',
'LH.BK',
'MINT.BK',
'MTC.BK',
'OR.BK',
'OSP.BK',
'PTT.BK',
'PTTEP.BK',
'PTTGC.BK',
'RATCH.BK',
'SAWAD.BK',
'SCB.BK',
'SCC.BK',
'SCGP.BK',
'TIDLOR.BK',
'TISCO.BK',
'TOP.BK',
'TRUE.BK',
'TTB.BK',
'TU.BK']
Download data from the symbol ticker list:
data = yf.download(symbol_list_set50, period='2y' , interval='1d')
[*********************100%***********************] 50 of 50 completed
data
Adj Close | … | Volume | |||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
ADVANC.BK | AOT.BK | AWC.BK | BANPU.BK | BBL.BK | BDMS.BK | BEM.BK | BGRIM.BK | BH.BK | BTS.BK | … | SAWAD.BK | SCB.BK | SCC.BK | SCGP.BK | TIDLOR.BK | TISCO.BK | TOP.BK | TRUE.BK | TTB.BK | TU.BK | |
Date | |||||||||||||||||||||
2021-04-20 | 162.436569 | 65.75 | 4.849284 | 10.588833 | 120.301224 | 21.133194 | 7.772137 | 43.162712 | 134.863342 | 8.486716 | … | 85769200 | NaN | 3327400 | 40092000 | NaN | 18187300 | 9110200 | 114465400.0 | 435867400 | 19536700 |
2021-04-21 | 166.149414 | 64.75 | 4.789417 | 10.502039 | 121.744835 | 20.941076 | 7.723862 | 43.409363 | 133.900024 | 8.440084 | … | 19975800 | NaN | 5057400 | 11496600 | NaN | 11952800 | 8884500 | 79843700.0 | 304208200 | 50532200 |
2021-04-22 | 162.900665 | 63.75 | 4.749505 | 10.328451 | 118.857605 | 20.652897 | 7.723862 | 43.409363 | 131.010086 | 8.393455 | … | 44729100 | NaN | 6497300 | 7890900 | NaN | 7348900 | 9271500 | 73508100.0 | 699419500 | 31829200 |
2021-04-23 | 161.508377 | 63.00 | 4.669681 | 10.328451 | 116.932785 | 21.037134 | 7.772137 | 42.176140 | 131.973389 | 8.393455 | … | 25841800 | NaN | 2940000 | 22495000 | NaN | 7459900 | 6146000 | 56871900.0 | 594705600 | 27583200 |
2021-04-26 | 160.116058 | 63.50 | 4.529990 | 10.154864 | 116.451584 | 21.421373 | 7.723862 | 41.682846 | 134.381683 | 8.300195 | … | 21250300 | NaN | 3529400 | 4658800 | NaN | 7776300 | 8181000 | 33715300.0 | 511760300 | 14662800 |
… | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … |
2023-04-12 | 211.000000 | 72.50 | 5.600000 | 9.950000 | 157.000000 | 29.500000 | 9.000000 | 40.000000 | 234.000000 | 7.600000 | … | 6377800 | 19913600.0 | 1224500 | 7742600 | 8876900.0 | 6382900 | 4457900 | 0.0 | 313801300 | 25480700 |
2023-04-17 | 213.000000 | 72.75 | 5.600000 | 9.800000 | 159.000000 | 30.250000 | 9.050000 | 40.000000 | 237.000000 | 7.750000 | … | 7464500 | 17896200.0 | 1280800 | 14120900 | 11467500.0 | 10881600 | 6274000 | 0.0 | 219106300 | 59864400 |
2023-04-18 | 212.000000 | 72.50 | 5.450000 | 9.600000 | 160.000000 | 30.000000 | 9.050000 | 39.750000 | 243.000000 | 7.700000 | … | 4400000 | 6897700.0 | 1004400 | 8190700 | 13660087.0 | 7862600 | 11900200 | 0.0 | 232123500 | 50972300 |
2023-04-19 | 212.000000 | 72.50 | 5.350000 | 9.600000 | 158.000000 | 29.500000 | 9.050000 | 39.250000 | 243.000000 | 7.700000 | … | 14278700 | 9688200.0 | 2447900 | 10146100 | 23555200.0 | 19367400 | 14185400 | 0.0 | 154405200 | 26477600 |
2023-04-20 | 210.000000 | 72.25 | 5.350000 | 9.500000 | 158.500000 | 29.500000 | 8.900000 | 38.500000 | 242.000000 | 7.600000 | … | 4122576 | 37657462.0 | 1841381 | 5902257 | 13315404.0 | 11325175 | 16190521 | 29574578.0 | 591730403 | 26221271 |
486 rows × 300 columns
Clean data and set round to 2:
data.fillna(method='ffill',inplace=True)
df = data.Close
df= np.round(df,2)
df
ADVANC.BK | AOT.BK | AWC.BK | BANPU.BK | BBL.BK | BDMS.BK | BEM.BK | BGRIM.BK | BH.BK | BTS.BK | … | SAWAD.BK | SCB.BK | SCC.BK | SCGP.BK | TIDLOR.BK | TISCO.BK | TOP.BK | TRUE.BK | TTB.BK | TU.BK | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Date | |||||||||||||||||||||
2021-04-20 | 175.0 | 65.75 | 4.86 | 12.20 | 127.5 | 22.00 | 8.05 | 43.75 | 140.0 | 9.10 | … | 88.25 | NaN | 418.0 | 50.00 | NaN | 101.00 | 55.25 | 3.32 | 1.20 | 14.7 |
2021-04-21 | 179.0 | 64.75 | 4.80 | 12.10 | 126.5 | 21.80 | 8.00 | 44.00 | 139.0 | 9.05 | … | 88.25 | NaN | 422.0 | 49.75 | NaN | 102.00 | 54.25 | 3.36 | 1.21 | 15.3 |
2021-04-22 | 175.5 | 63.75 | 4.76 | 11.90 | 123.5 | 21.50 | 8.00 | 44.00 | 136.0 | 9.00 | … | 83.50 | NaN | 430.0 | 49.50 | NaN | 101.00 | 54.00 | 3.32 | 1.19 | 14.9 |
2021-04-23 | 174.0 | 63.00 | 4.68 | 11.90 | 121.5 | 21.90 | 8.05 | 42.75 | 137.0 | 9.00 | … | 82.00 | NaN | 428.0 | 49.25 | NaN | 101.00 | 53.50 | 3.30 | 1.16 | 14.8 |
2021-04-26 | 172.5 | 63.50 | 4.54 | 11.70 | 121.0 | 22.30 | 8.00 | 42.25 | 139.5 | 8.90 | … | 84.25 | NaN | 434.0 | 49.50 | NaN | 101.00 | 53.75 | 3.32 | 1.17 | 14.8 |
… | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … |
2023-04-12 | 211.0 | 72.50 | 5.60 | 9.95 | 157.0 | 29.50 | 9.00 | 40.00 | 234.0 | 7.60 | … | 56.50 | 105.50 | 316.0 | 44.75 | 26.50 | 101.50 | 52.50 | 8.40 | 1.40 | 13.3 |
2023-04-17 | 213.0 | 72.75 | 5.60 | 9.80 | 159.0 | 30.25 | 9.05 | 40.00 | 237.0 | 7.75 | … | 56.75 | 101.50 | 315.0 | 43.75 | 26.50 | 102.00 | 52.00 | 8.40 | 1.40 | 13.7 |
2023-04-18 | 212.0 | 72.50 | 5.45 | 9.60 | 160.0 | 30.00 | 9.05 | 39.75 | 243.0 | 7.70 | … | 57.00 | 101.00 | 312.0 | 44.00 | 23.78 | 101.50 | 50.50 | 8.40 | 1.42 | 14.1 |
2023-04-19 | 212.0 | 72.50 | 5.35 | 9.60 | 158.0 | 29.50 | 9.05 | 39.25 | 243.0 | 7.70 | … | 55.75 | 100.50 | 309.0 | 43.50 | 23.20 | 99.75 | 49.50 | 8.40 | 1.42 | 14.1 |
2023-04-20 | 210.0 | 72.25 | 5.35 | 9.50 | 158.5 | 29.50 | 8.90 | 38.50 | 242.0 | 7.60 | … | 56.00 | 99.75 | 305.0 | 43.50 | 22.90 | 99.75 | 48.75 | 8.00 | 1.42 | 13.8 |
486 rows × 50 columns
Calculating the daily percentage change of the stock prices using the pct_change() function. Then, we apply the np.sign() function to determine whether the change is positive (advancing), negative (declining), or zero (unchanged):
np.sign(df.pct_change())
ADVANC.BK | AOT.BK | AWC.BK | BANPU.BK | BBL.BK | BDMS.BK | BEM.BK | BGRIM.BK | BH.BK | BTS.BK | … | SAWAD.BK | SCB.BK | SCC.BK | SCGP.BK | TIDLOR.BK | TISCO.BK | TOP.BK | TRUE.BK | TTB.BK | TU.BK | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Date | |||||||||||||||||||||
2021-04-20 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | … | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
2021-04-21 | 1.0 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | 1.0 | -1.0 | -1.0 | … | 0.0 | NaN | 1.0 | -1.0 | NaN | 1.0 | -1.0 | 1.0 | 1.0 | 1.0 |
2021-04-22 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | 0.0 | 0.0 | -1.0 | -1.0 | … | -1.0 | NaN | 1.0 | -1.0 | NaN | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 |
2021-04-23 | -1.0 | -1.0 | -1.0 | 0.0 | -1.0 | 1.0 | 1.0 | -1.0 | 1.0 | 0.0 | … | -1.0 | NaN | -1.0 | -1.0 | NaN | 0.0 | -1.0 | -1.0 | -1.0 | -1.0 |
2021-04-26 | -1.0 | 1.0 | -1.0 | -1.0 | -1.0 | 1.0 | -1.0 | -1.0 | 1.0 | -1.0 | … | 1.0 | NaN | 1.0 | 1.0 | NaN | 0.0 | 1.0 | 1.0 | 1.0 | 0.0 |
… | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … | … |
2023-04-12 | -1.0 | -1.0 | 0.0 | -1.0 | -1.0 | -1.0 | 1.0 | 0.0 | -1.0 | 1.0 | … | 1.0 | -1.0 | 1.0 | -1.0 | -1.0 | 1.0 | -1.0 | 0.0 | -1.0 | -1.0 |
2023-04-17 | 1.0 | 1.0 | 0.0 | -1.0 | 1.0 | 1.0 | 1.0 | 0.0 | 1.0 | 1.0 | … | 1.0 | -1.0 | -1.0 | -1.0 | 0.0 | 1.0 | -1.0 | 0.0 | 0.0 | 1.0 |
2023-04-18 | -1.0 | -1.0 | -1.0 | -1.0 | 1.0 | -1.0 | 0.0 | -1.0 | 1.0 | -1.0 | … | 1.0 | -1.0 | -1.0 | 1.0 | -1.0 | -1.0 | -1.0 | 0.0 | 1.0 | 1.0 |
2023-04-19 | 0.0 | 0.0 | -1.0 | 0.0 | -1.0 | -1.0 | 0.0 | -1.0 | 0.0 | 0.0 | … | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | -1.0 | 0.0 | 0.0 | 0.0 |
2023-04-20 | -1.0 | -1.0 | 0.0 | -1.0 | 1.0 | 0.0 | -1.0 | -1.0 | -1.0 | -1.0 | … | 1.0 | -1.0 | -1.0 | 0.0 | -1.0 | 0.0 | -1.0 | -1.0 | 0.0 | -1.0 |
486 rows × 50 columns
np.sign(df.pct_change()).stack()
Date
2021-04-21 ADVANC.BK 1.0
AOT.BK -1.0
AWC.BK -1.0
BANPU.BK -1.0
BBL.BK -1.0
...
2023-04-20 TISCO.BK 0.0
TOP.BK -1.0
TRUE.BK -1.0
TTB.BK 0.0
TU.BK -1.0
Length: 23996, dtype: float64
np.sign(df.pct_change()).stack().groupby(level=[0]).value_counts()
Date
2021-04-21 -1.0 24
1.0 16
0.0 8
2021-04-22 -1.0 36
0.0 8
..
2023-04-19 0.0 13
1.0 2
2023-04-20 -1.0 37
0.0 8
1.0 5
Length: 1450, dtype: int64
After processing the data, we stack it and group it by date using the groupby() function. The value_counts() function is used to count the occurrences of each sign (advancers, decliners, and unchanged) for each date:
np.sign(df.pct_change()).stack().groupby(level=[0]).value_counts().unstack()
-1.0 | 0.0 | 1.0 | |
---|---|---|---|
Date | |||
2021-04-21 | 24.0 | 8.0 | 16.0 |
2021-04-22 | 36.0 | 8.0 | 4.0 |
2021-04-23 | 33.0 | 9.0 | 6.0 |
2021-04-26 | 19.0 | 9.0 | 20.0 |
2021-04-27 | 19.0 | 11.0 | 18.0 |
… | … | … | … |
2023-04-12 | 27.0 | 7.0 | 16.0 |
2023-04-17 | 11.0 | 10.0 | 29.0 |
2023-04-18 | 29.0 | 8.0 | 13.0 |
2023-04-19 | 35.0 | 13.0 | 2.0 |
2023-04-20 | 37.0 | 8.0 | 5.0 |
485 rows × 3 columns
Next, we create a new DataFrame called breadth_df to store the results and rename the columns to ‘decliners’, ‘unchanged’, and ‘advancers’:
breadth_df = np.sign(df.pct_change()).stack().groupby(level=[0]).value_counts().unstack()
breadth_df.columns=['decliners','unchanged','advancers']
Calculate the ADR by dividing the number of advancers by the number of decliners:
# Calculate ADR (ADvancer/Decliner)
breadth_df['ADR']=breadth_df['advancers']/breadth_df['decliners']
To prepare the data for visualization, we reset the index of the DataFrame:
breadth_df1=breadth_df.reset_index()
breadth_df1
Date | decliners | unchanged | advancers | ADR | |
---|---|---|---|---|---|
0 | 2021-04-21 | 24.0 | 8.0 | 16.0 | 0.666667 |
1 | 2021-04-22 | 36.0 | 8.0 | 4.0 | 0.111111 |
2 | 2021-04-23 | 33.0 | 9.0 | 6.0 | 0.181818 |
3 | 2021-04-26 | 19.0 | 9.0 | 20.0 | 1.052632 |
4 | 2021-04-27 | 19.0 | 11.0 | 18.0 | 0.947368 |
… | … | … | … | … | … |
480 | 2023-04-12 | 27.0 | 7.0 | 16.0 | 0.592593 |
481 | 2023-04-17 | 11.0 | 10.0 | 29.0 | 2.636364 |
482 | 2023-04-18 | 29.0 | 8.0 | 13.0 | 0.448276 |
483 | 2023-04-19 | 35.0 | 13.0 | 2.0 | 0.057143 |
484 | 2023-04-20 | 37.0 | 8.0 | 5.0 | 0.135135 |
485 rows × 5 columns
Finally, we use Plotly Express to create a bar chart that plots the ADR over time:
import plotly.express as px
fig = px.bar(breadth_df1,y='ADR',x='Date',text='ADR',template='simple_white',title='Market Breadth :Advance /Decline Ratio')
fig.update_traces(texttemplate='%{text:.2s}',textposition='outside')
fig.update_traces(textfont_size=28,textangle=0,textposition='outside',cliponaxis=False)
fig.update_xaxes(rangebreaks=[dict(bounds=['sat','mon'])])
fig.update_xaxes(rangeslider_visible=True)
fig.show()